As one of the first PHP frameworks created, CakePHP is among the most popular PHP frameworks today. CakePHP, being a very simple, open-source and rapid development framework, helps to build web applications much faster. It is an open-source MVC framework written in PHP, modeled after the concepts of Ruby on Rails that provides an extensible architecture for developing, maintaining, and deploying web applications.
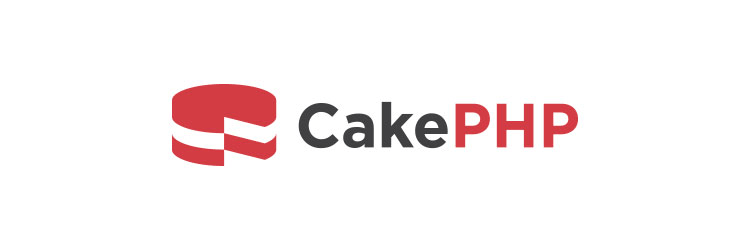
CakePHP enforces conventions at the macro and micro levels.
At the macro level, it follows the model-view-controller (MVC) architectural pattern.
This is a way of organizing the code for an application into three core components:
- the model, which defines how data is organized
- the view, which defines how data is displayed
- the controller, which defines how data is managed and manipulated.
At the micro-level, CakePHP has conventions for everything from naming things, to the folder structure, to URL capitalization.
It is possible to ignore some of these conventions, but there usually isn’t any compelling reason to do so.
And if you do follow these conventions, a number of things happen automagically.
If you follow the conventions properly, CakePHP getting your model classes, your view templates, your database tables, and your URL structure to cooperate becomes a trivial matter.
Since you don’t have to re-invent the wheel for each new data type, you can focus on the problem you are trying to solve and get things done faster.
Another way that CakePHP helps speed up web development is through its command-line tool.
This gives you the ability to quickly spin up a new application skeleton, add new models and views, launch build processes, run tests, and manage deployment.
Features of Cake PHP
Following are some of the important features that make Cake PHP the first choice of software architects
Object-Relational Mapping
Object-relational mapping is a programming technique to facilitate data conversion between incompatible type systems in databases and OOPs. That means every table is represented by a class. These classes are responsible for the management of whatever happens with your data, its validity, interactions, and evolution of the information workflow in your domain of work. The practice blends so well with CakePHP development that you would go on writing the whole program without even writing a single line of SQL code.
User-Friendly Extensions:
If you are using CakePHP framework, you will easily extend your project with components, behaviors, and plug-ins. It can create parts of the reusable code that becomes useful for multiple projects. By expanding the functionality through these components, behaviors, and plug-ins, you will create a generalized library. This library can share between different models, controllers, and views. As well as, it can be used in other projects. Moreover, there are multiple plug-ins, helpers, and components available for CakePHP users that are developed by well-defined conventions of CakePHP so you don’t have to write everything from scratch.
MVC Pattern:
Model View Controller targets modern programming needs. CakePHP uses it just right with all the entities working together excellently and providing a great way to work on different aspects of development in a vitally planned system of data processing. The model supports data handling, view supports data rendering, controller dynamically responds to events to produce a right functionary for the framework and that’s how you get the best way to interact with the database and other important facets of solution where data plays an important part.
Example of creating a Form Model
The model will be found in /app/ models/form.php
<?php class Form extends AppModel { #Define the name of the Model var $name = 'Form'; } ?>
Example of creating a Forms View
The view will be found in /app/views/forms/index.ctp
<div id="form"> <table> <tr> <th>Id</th> <th>Data</th> </tr> #Here we loop through the $data array that we have defined in our controller <?php foreach ($data as $d) { ?> <tr> <td><?= $d['Form']['id'] ?></td> <td><?= $d['Form']['data'] ?></td> </tr> <?php } ?> </table> <?php #Create a form using CakePHP’s integrated Core Helpers and define the action of the controller, which points the form to the page it will be posted to, through the options array. echo $form->create('Form', array('action' => 'index')); #Create the input using the Core Helpers again. The ‘type’ defines the type of the input field and the ‘label’ defines what will be written above the input. echo $form->input('data', array( 'type' => 'text', 'label' => 'Add new entry:' )); #Create a submit button and a </form> tag. echo $form->end('Submit'); ?> </div>
Example of creating a Forms Controller
The controller will be found in /app/controllers/forms_controller.php.
<?php class FormsController extends AppController { #Define the name of the controller var $name = 'Forms'; #Define which model we will be using. This, by convention is Form and if our model is Form, then we can skip it. var $uses = array('Form'); // This is the function that will be used when http://domain.com/CakePHP/forms/index is accessed function index() { #Check the ‘$this->data’ variable to see if something is submitted to the forms controller that uses the Form model. if(!empty($this->data['Form'])) { #The $this->Form->save() method saves everything that is submitted to the database if($this->Form->save($this->data['Form'])) { #Display a confirmation message and redirect to the forms controller after 2 seconds $this->flash('The form was successfully submitted.<br>You will be redirected within 2 seconds..','/forms/','2'); } } #The $this->Form->find(‘all’) method will get all the data from the database and assign it to the variable $data. $this->set('data', $this->Form->find('all')); } } ?>
Easy Configurations
You just don’t need to specify or signal anything to establish any connection between the different system resources and don’t need to configure them at all. Everything with CakePHP runs on auto-detect and hence you don’t need to care about any sort of installation or fixing anywhere at any level.
Tools
- Packages is the official directory of plugins, modules, extensions, and other tools for CakePHP.
- Turnkey Linux provides a rapid-deployment Linux image with CakePHP and all needed dependencies, along with easy tools for running on various virtual machines and deploying to Amazon.
- CakePHP Tools is a CakePHP plugin containing lots of useful and reusable tools.
- Awesome CakePHP is a curated list of the best and most interesting tools for CakePHP.